Hi @tsiaraf,
just use the python api for things like this.
Here is an example.
First we create some geometry and mesh it.
#!cubit
reset
create cylinder r 1 height 10
vol 1 size auto factor 6
mesh vol 1
As the python api doesn’t have a function to get the centroid directly for a element, we will define us one.
#!python
def get_centroid(el_type,id):
node_ids = cubit.get_connectivity(el_type,id)
centroid = [0,0,0]
for id in node_ids:
coord = cubit.get_nodal_coordinates(id)
centroid[0] += coord[0]
centroid[1] += coord[1]
centroid[2] += coord[2]
centroid[0] = round(centroid[0]/len(node_ids),6)
centroid[1] = round(centroid[1]/len(node_ids),6)
centroid[2] = round(centroid[2]/len(node_ids),6)
return centroid
Then we just need to query the hex ids for our volume
hex_ids = cubit.parse_cubit_list("hex","all")
When we have the ids we just make a loop and write the info into a file.
file = open("example.txt", "w")
for id in hex_ids:
centroid = get_centroid("hex",id)
file.write(f"hex id: {id} centroid x: {centroid[0]} y: {centroid[1]} z: {centroid[2]} \n")
file.close()
In your current working directory you should now find the example.txt with your requested data in it.
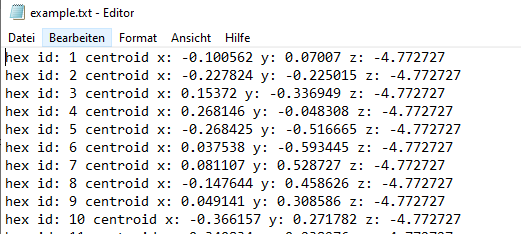
And here is the full input again, you just need to copy/paste and run it.
#!cubit
reset
create cylinder r 1 height 10
vol 1 size auto factor 6
mesh vol 1
#!python
def get_centroid(el_type,id):
node_ids = cubit.get_connectivity(el_type,id)
centroid = [0,0,0]
for id in node_ids:
coord = cubit.get_nodal_coordinates(id)
centroid[0] += coord[0]
centroid[1] += coord[1]
centroid[2] += coord[2]
centroid[0] = round(centroid[0]/len(node_ids),6)
centroid[1] = round(centroid[1]/len(node_ids),6)
centroid[2] = round(centroid[2]/len(node_ids),6)
return centroid
hex_ids = cubit.parse_cubit_list("hex","all")
file = open("example.txt", "w")
for id in hex_ids:
centroid = get_centroid("hex",id)
file.write(f"hex id: {id} centroid x: {centroid[0]} y: {centroid[1]} z: {centroid[2]} \n")
file.close()